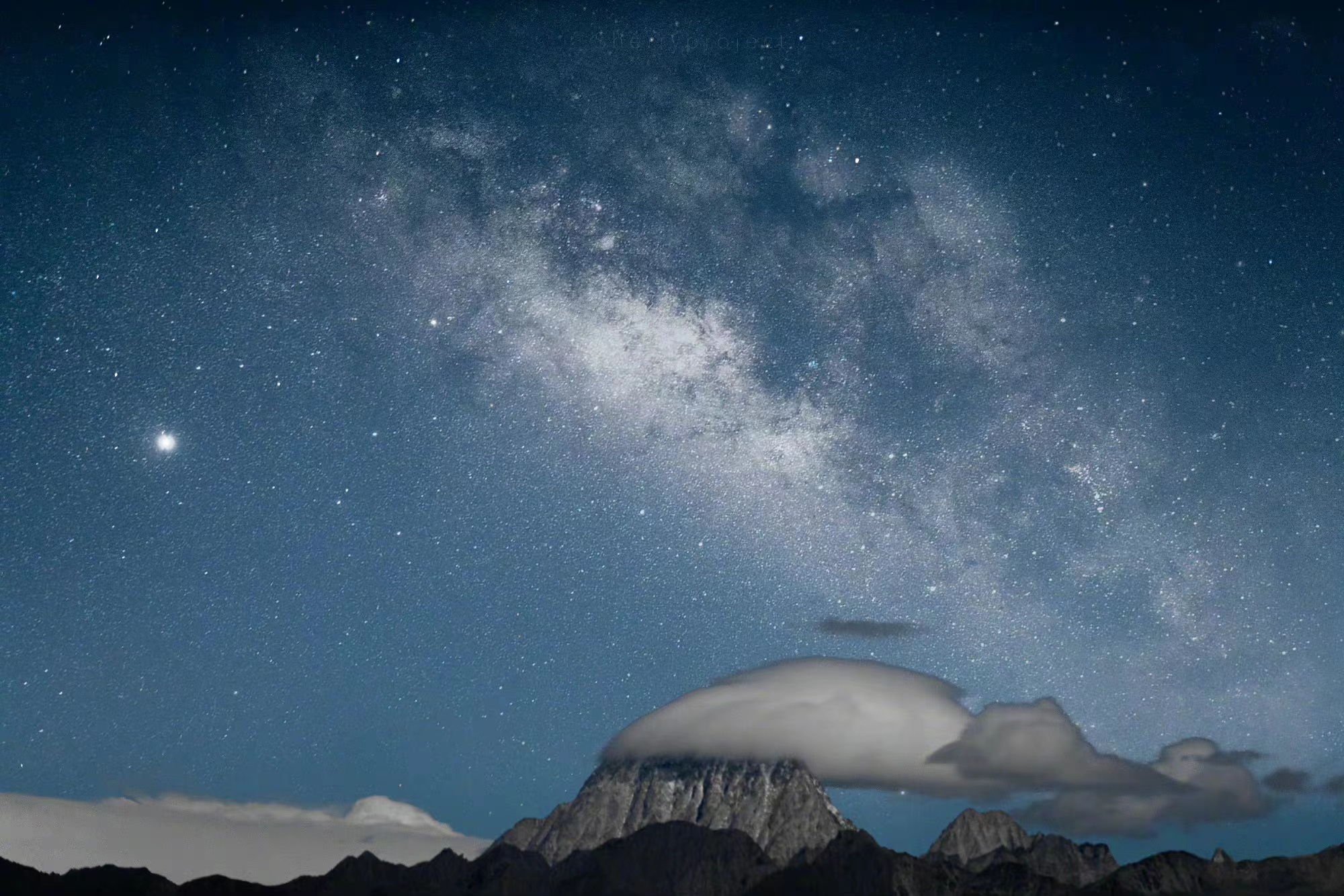
un7.22:如何在IDEA和VS-Code中用若依框架同时实现视频和图片的上传?
随着若依框架的便利性,越来越多的程序员选择使用,随之而来的也就有各种各样的问题出现,那么我们应该如何使用若依框架同时实现图片和视频上传的功能呢?其实这和我7.7写的那篇文章大同小异。接下来,我就给大家分享这个过程。......
随着若依框架的便利性,越来越多的程序员选择使用,随之而来的也就有各种各样的问题出现,那么我们应该如何使用若依框架同时实现图片和视频上传的功能呢?其实这和我7.7写的那篇文章大同小异。接下来,我就给大家分享这个过程。
所需软件:前端:VS-Code。后端:IDEA。
一、在ruoyi-admin下创建一个commons包,创建一个实体包,在entity下创建一个类,我的名字叫FileInfo。
package com.ruoyi.commons.entity;
public class FileInfo {
private String fileName;
private boolean isSecret;
private String absolutPath;
private String relativePath;
public String getFileName() {
return fileName;
}
public void setFileName(String fileName) {
this.fileName = fileName;
}
public String getAbsolutPath() {
return absolutPath;
}
public void setAbsolutPath(String absolutPath) {
this.absolutPath = absolutPath;
}
public String getRelativePath() {
return relativePath;
}
public void setRelativePath(String relativePath) {
this.relativePath = relativePath;
}
public boolean isSecret() {
return isSecret;
}
public void setSecret(boolean isSecret) {
this.isSecret = isSecret;
}
public FileInfo() {
}
public FileInfo(String fileName, boolean isSecret, String absolutPath, String relativePath) {
super();
this.fileName = fileName;
this.isSecret = isSecret;
this.absolutPath = absolutPath;
this.relativePath = relativePath;
}
@Override
public String toString() {
return "FileInfo [fileName=" + fileName + ", isSecret=" + isSecret + ", absolutPath=" + absolutPath
+ ", relativePath=" + relativePath + "]";
}
}
二、在ruoyi-system下也同样创建一个commons包,和刚才的步骤一样,将FileInfo类放入entity。
三、在commons包下创建一个cast类。
package com.ruoyi.commons;
/**
* 常量的定义,一般用于系统的配置参数
*/
public class Cast {
public static final String FILE_SERVER_URL = "http://127.0.0.1:8060";//文件服务器地址
}
四、在commons包下创建一个utils包,在utils包下创建一个JesyFileUploadUtil类,直接复制粘贴就可以,然后导导包。
package com.ruoyi.commons.utils;
import java.io.IOException;
import java.util.Date;
import java.util.Random;
import javax.net.ssl.SSLContext;
import com.ruoyi.commons.entity.FileInfo;
import org.springframework.web.multipart.MultipartFile;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.WebResource;
import com.sun.jersey.api.client.config.ClientConfig;
import com.sun.jersey.api.client.config.DefaultClientConfig;
import com.sun.jersey.client.urlconnection.HTTPSProperties;
/**
* 跨服务器文件上传工具类
*
* @author qin
*
*/
public class JesyFileUploadUtil {
/**
* 上传文件
*
* @param file --多媒体文件
* @param serverUrl --服务器路径http://127.0.0.1:8080/ssm_image_server
* @return
* @throws IOException
*/
public static FileInfo uploadFile(MultipartFile file, String serverUrl) throws IOException {
//把request请求转换成多媒体的请求对象
// MultipartHttpServletRequest mh = (MultipartHttpServletRequest) request;
//根据文件名获取文件对象
// MultipartFile cm = mh.getFile(fileName);
//获取文件的上传流
byte[] fbytes = file.getBytes();
//重新设置文件名
String newFileName = "";
newFileName += new Date().getTime()+""; //将当前时间获得的毫秒数拼接到新的文件名上
//随机生成一个3位的随机数
Random r = new Random();
for(int i=0; i<3; i++) {
newFileName += r.nextInt(10); //生成一个0-10之间的随机整数
}
//获取文件的扩展名
String orginalFilename = file.getOriginalFilename();
String suffix = orginalFilename.substring(orginalFilename.indexOf("."));
//创建jesy服务器,进行跨服务器上传
// Client client = Client.create(getClientConfig());
Client client = Client.create();
//把文件关联到远程服务器
//http://127.0.0.1:8080/ssm_image_server/upload/123131312321.jpg
WebResource resource = client.resource(serverUrl+"/upload/"+newFileName+suffix);
//上传
resource.put(String.class, fbytes);
//图片上传成功后要做的事儿
//1、ajax回调函数做图片回显(需要图片的完整路径)
//2、将图片的路径保存到数据库(需要图片的相对路径)
String fullPath = serverUrl+"/upload/"+newFileName+suffix; //全路径
String relativePath = "/upload/"+newFileName+suffix; //相对路径
// //生成一个json响应给客户端
// String resultJson = "{\"fullPath\":\""+fullPath+"\", \"relativePath\":\""+relativePath+"\"}";
// return resultJson;
return new FileInfo(newFileName+suffix, false, fullPath, relativePath);
}
}
五、给pom.xml文件中加入依赖,这里注意,是system下的pom文件,别加到整个项目了。
<!-- apache_ftp:Begin -->
<!-- httpclient begin -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
<!-- httpclient end -->
<!-- ftp begin -->
<dependency>
<groupId>commons-net</groupId>
<artifactId>commons-net</artifactId>
<version>3.5</version>
</dependency>
<!-- ftp end -->
<!-- apache_ftp:End -->
<!-- 文件上传:Begin -->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3.1</version>
</dependency>
<!-- 文件上传:End -->
<!--跨服务器上传:Begin -->
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-client</artifactId>
<version>1.19</version>
</dependency>
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-core</artifactId>
<version>1.19</version>
</dependency>
<!--跨服务器上传:End -->
六、service中声明方法。
/**
* 文件上传
* @param file
* @return
*/
public FileInfo upload(MultipartFile file);
七、实现类中实现此方法。
@Override
public FileInfo upload(MultipartFile file) {
FileInfo fileInfo = null;
try {
fileInfo = JesyFileUploadUtil.uploadFile(file, Cast.FILE_SERVER_URL);
} catch (IOException e) {
e.printStackTrace();
}
return fileInfo;
}
八、controller中直接创建接口,方便前端调用。
/**
* 照片上传
* @param picfile
* @return
*/
@PostMapping("pic_upload")
public AjaxResult uploadPic(@RequestParam("picfile")MultipartFile picfile){
FileInfo info = videoService.upload(picfile);
return AjaxResult.success(info);
}
/**
* 视频上传
* @param videofile
* @return
*/
@PostMapping("video_upload")
public AjaxResult uploadVideo(@RequestParam("videofile")MultipartFile videofile){
FileInfo info = videoService.upload(videofile);
return AjaxResult.success(info);
}
至此,后端代码完成,接下来,完成前端代码。
一、打开views文件下的videoindex.vue文件,在视图层的表单中写上传的代码。
<el-form-item label="视频" prop="videoWay">
<el-upload ref="pic" :headers="headers" :on-success="videoSuccess" :file-list="videofileList" :action="videoAction"
:before-upload="videoBeforeUpload" name="videofile" accept=".MP4">
<el-button size="small" type="primary" icon="el-icon-upload">点击上传</el-button>
<!-- <div slot="tip" class="el-upload__tip">只能上传不超过200KB的图片文件</div> -->
</el-upload>
</el-form-item>
<el-form-item label="封面图片" prop="videoAddress">
<el-upload ref="pic" :headers="headers" :on-success="urlSuccess" :file-list="videofileList" :action="picAction"
:before-upload="videoBeforeUpload" name="picfile" accept=".jpg">
<el-button size="small" type="primary" icon="el-icon-upload">点击上传</el-button>
<!-- <div slot="tip" class="el-upload__tip">只能上传不超过200KB的图片文件</div> -->
</el-upload>
</el-form-item>
二、在业务层引进getToken。
import { getToken } from '@/utils/auth';
三、将headers中的数据进行接收并声明,然后将参数进行声明,路径一定要写对。
data(){
return{
headers:{
Authorization:"Bearer " + getToken()
},
videofileList:[],
videoAction: process.env.VUE_APP_BASE_API + "/basic/video/video_upload",
picAction: process.env.VUE_APP_BASE_API + "/basic/video/pic_upload",
四、在methods中写对应函数。
/**
* 视频上传前的处理
*/
videoBeforeUpload(file){
return true;
},
/**
* 视频上传之后的回调
*/
videoSuccess(response,file, fileList){
console.log(response);
},
/**
* 图片上传前的处理
*/
videoBeforeUpload(file){
return true;
},
/**
* 图片上传之后的回调
*/
urlSuccess(response,file, fileList){
console.log(response);
},
至此,前端代码完成,接下来,进行测试。
一、打开tomcat服务器,bin中的startup,则显示如下图片。
二、将项目全部启动。
1、测试前。
2、测试后。
如此,我们便在若依框架中实现了同时将图片和视频上传的功能了。
最后,附上完整代码。
FileInfo
package com.ruoyi.commons.entity;
public class FileInfo {
private String fileName;
private boolean isSecret;
private String absolutPath;
private String relativePath;
public String getFileName() {
return fileName;
}
public void setFileName(String fileName) {
this.fileName = fileName;
}
public String getAbsolutPath() {
return absolutPath;
}
public void setAbsolutPath(String absolutPath) {
this.absolutPath = absolutPath;
}
public String getRelativePath() {
return relativePath;
}
public void setRelativePath(String relativePath) {
this.relativePath = relativePath;
}
public boolean isSecret() {
return isSecret;
}
public void setSecret(boolean isSecret) {
this.isSecret = isSecret;
}
public FileInfo() {
}
public FileInfo(String fileName, boolean isSecret, String absolutPath, String relativePath) {
super();
this.fileName = fileName;
this.isSecret = isSecret;
this.absolutPath = absolutPath;
this.relativePath = relativePath;
}
@Override
public String toString() {
return "FileInfo [fileName=" + fileName + ", isSecret=" + isSecret + ", absolutPath=" + absolutPath
+ ", relativePath=" + relativePath + "]";
}
}
cast
package com.ruoyi.commons;
/**
* 常量的定义,一般用于系统的配置参数
*/
public class Cast {
public static final String FILE_SERVER_URL = "http://127.0.0.1:8060";//文件服务器地址
}
JesyFileUploadUtil
package com.ruoyi.commons.utils;
import java.io.IOException;
import java.util.Date;
import java.util.Random;
import javax.net.ssl.SSLContext;
import com.ruoyi.commons.entity.FileInfo;
import org.springframework.web.multipart.MultipartFile;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.WebResource;
import com.sun.jersey.api.client.config.ClientConfig;
import com.sun.jersey.api.client.config.DefaultClientConfig;
import com.sun.jersey.client.urlconnection.HTTPSProperties;
/**
* 跨服务器文件上传工具类
*
* @author qin
*
*/
public class JesyFileUploadUtil {
/**
* 上传文件
*
* @param file --多媒体文件
* @param serverUrl --服务器路径http://127.0.0.1:8080/ssm_image_server
* @return
* @throws IOException
*/
public static FileInfo uploadFile(MultipartFile file, String serverUrl) throws IOException {
//把request请求转换成多媒体的请求对象
// MultipartHttpServletRequest mh = (MultipartHttpServletRequest) request;
//根据文件名获取文件对象
// MultipartFile cm = mh.getFile(fileName);
//获取文件的上传流
byte[] fbytes = file.getBytes();
//重新设置文件名
String newFileName = "";
newFileName += new Date().getTime()+""; //将当前时间获得的毫秒数拼接到新的文件名上
//随机生成一个3位的随机数
Random r = new Random();
for(int i=0; i<3; i++) {
newFileName += r.nextInt(10); //生成一个0-10之间的随机整数
}
//获取文件的扩展名
String orginalFilename = file.getOriginalFilename();
String suffix = orginalFilename.substring(orginalFilename.indexOf("."));
//创建jesy服务器,进行跨服务器上传
// Client client = Client.create(getClientConfig());
Client client = Client.create();
//把文件关联到远程服务器
//http://127.0.0.1:8080/ssm_image_server/upload/123131312321.jpg
WebResource resource = client.resource(serverUrl+"/upload/"+newFileName+suffix);
//上传
resource.put(String.class, fbytes);
//图片上传成功后要做的事儿
//1、ajax回调函数做图片回显(需要图片的完整路径)
//2、将图片的路径保存到数据库(需要图片的相对路径)
String fullPath = serverUrl+"/upload/"+newFileName+suffix; //全路径
String relativePath = "/upload/"+newFileName+suffix; //相对路径
// //生成一个json响应给客户端
// String resultJson = "{\"fullPath\":\""+fullPath+"\", \"relativePath\":\""+relativePath+"\"}";
// return resultJson;
return new FileInfo(newFileName+suffix, false, fullPath, relativePath);
}
}
service
package com.ruoyi.basic.service;
import com.ruoyi.basic.domain.Video;
import com.ruoyi.commons.entity.FileInfo;
import org.springframework.web.multipart.MultipartFile;
import java.util.List;
public interface IVideoService {
/**
* 文件上传
* @param file
* @return
*/
public FileInfo upload(MultipartFile file);
/**
* 查询视频列表
* @param video 视频信息
* @return 视频集合
*/
public List<Video> selectVideoList(Video video);
/**
* 新增视频
* @param video
* @return
*/
public int insertVideo(Video video);
/**
* 删除视频
* @param id
* @return
*/
public int deleteVideoById(String id);
/**
* 根据视频ID查询视频信息
* @param id
* @return
*/
public Video selectVideoById(String id);
/**
* 修改视频
* @param video
* @return
*/
public int updateVideo(Video video);
}
serviceImpl
package com.ruoyi.basic.service.impl;
import com.ruoyi.basic.domain.Video;
import com.ruoyi.basic.mapper.VideoMapper;
import com.ruoyi.basic.service.IVideoService;
import com.ruoyi.commons.Cast;
import com.ruoyi.commons.entity.FileInfo;
import com.ruoyi.commons.utils.JesyFileUploadUtil;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.util.List;
import java.util.UUID;
@Service
public class VideoServiceImpl implements IVideoService {
@Autowired(required = false)
private VideoMapper videoMapper;
@Override
public FileInfo upload(MultipartFile file) {
FileInfo fileInfo = null;
try {
fileInfo = JesyFileUploadUtil.uploadFile(file, Cast.FILE_SERVER_URL);
} catch (IOException e) {
e.printStackTrace();
}
return fileInfo;
}
@Override
public List<Video> selectVideoList(Video video) {
return videoMapper.selectVideoList(video);
}
@Override
public int insertVideo(Video video) {
String id= UUID.randomUUID().toString().replace("-","");
video.setId(id);
return videoMapper.insertVideo(video);
}
@Override
public int deleteVideoById(String id) {
return videoMapper.deleteVideoById(id);
}
@Override
public Video selectVideoById(String id) {
return videoMapper.selectVideoById(id);
}
@Override
public int updateVideo(Video video) {
return videoMapper.updateVideo(video);
}
}
controller
package com.ruoyi.basic.controller;
import com.ruoyi.basic.domain.Video;
import com.ruoyi.basic.service.IVideoService;
import com.ruoyi.common.core.controller.BaseController;
import com.ruoyi.common.core.domain.AjaxResult;
import com.ruoyi.common.core.page.TableDataInfo;
import com.ruoyi.commons.entity.FileInfo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import java.util.List;
@RestController
@RequestMapping("/basic/video")
public class VideoController extends BaseController {
@Autowired(required = false)
private IVideoService videoService;
@GetMapping("/list")
public TableDataInfo list(Video video){
startPage();
List<Video> videos = videoService.selectVideoList(video);
return getDataTable(videos);
}
@PostMapping("/add")
public AjaxResult add(Video video){
int rows=videoService.insertVideo(video);
return toAjax(rows);
}
@PostMapping("/remove")
public AjaxResult remove(String id){
int rows=videoService.deleteVideoById(id);
return toAjax(rows);
}
@GetMapping("/get_info")
public AjaxResult getInfo(String id){
return AjaxResult.success(videoService.selectVideoById(id));
}
@GetMapping("/edit")
public AjaxResult edit(Video video){
int rows=videoService.updateVideo(video);
return toAjax(rows);
}
/**
* 照片上传
* @param picfile
* @return
*/
@PostMapping("pic_upload")
public AjaxResult uploadPic(@RequestParam("picfile")MultipartFile picfile){
FileInfo info = videoService.upload(picfile);
return AjaxResult.success(info);
}
/**
* 视频上传
* @param videofile
* @return
*/
@PostMapping("video_upload")
public AjaxResult uploadVideo(@RequestParam("videofile")MultipartFile videofile){
FileInfo info = videoService.upload(videofile);
return AjaxResult.success(info);
}
}
videoindex
<template>
<div class="app-container">
<el-form :model="queryParam" ref="queryForm" size="small" :inline="true" label-width="68px">
<el-form-item label="所属病区" prop="wardId">
<el-select v-model="queryParam.wardId" placeholder="请选择" size="small">
<el-option v-for="s in listWard" :label="s.wardName" :value="s.id" :key="s.id">
</el-option>
</el-select>
</el-form-item>
<el-form-item>
<el-button type="primary" size="mini" icon="el-icon-search" @click="handelQuery">搜索</el-button>
<el-button size="mini" icon="el-icon-refresh" @click="resetQuery">重置</el-button>
</el-form-item>
</el-form>
<el-row :gutter="10" >
<el-col >
<el-button plain @click="handleAdd" type="primary" icon="el-icon-plus" size="mini">
新增
</el-button>
</el-col>
</el-row>
<el-table :data="videoList">
<el-table-column label="ID" prop="id" align="center" ></el-table-column>
<el-table-column label="视频名称" prop="video" align="center" ></el-table-column>
<el-table-column label="所属病区" prop="wardName" align="center" ></el-table-column>
<el-table-column label="路径" prop="videoWay" align="center" ></el-table-column>
<el-table-column label="封面图片地址" prop="videoAddress" align="center" ></el-table-column>
<el-table-column label="视频排序" prop="videoSort" align="center" ></el-table-column>
<el-table-column label="操作" align="center">
<template slot-scope="scope">
<el-button @click="handleUpdate(scope.row)" type="text" size="mini" icon="el-icon-edit" >修改</el-button>
<el-button @click="handledelecte(scope.row)" type="text" size="mini" icon="el-icon-delete">删除</el-button>
</template>
</el-table-column>
</el-table>
<!-- 分页查询 -->
<pagination
:total="queryParam.total"
:page.sync="queryParam.pageNum"
:limit.sync="queryParam.pageSize"
@pagination="getList"
/>
<!-- 对话框 -->
<el-dialog :title="title" width="500px" :visible.sync="open" append-to-body>
<!-- 表单 -->
<el-form ref="form" :model="form" label-width="80px">
<el-form-item label="视频名称" prop="video">
<el-input v-model="form.video" placeholder="请输入您要添加的视频名"></el-input>
</el-form-item>
<el-form-item label="所属病区" prop="wardId">
<el-select v-model="queryParam.wardId" placeholder="请选择" size="small">
<el-option v-for="s in listWard" :label="s.wardName" :value="s.id" :key="s.id">
</el-option>
</el-select>
</el-form-item>
<el-form-item label="视频" prop="videoWay">
<el-upload ref="pic" :headers="headers" :on-success="videoSuccess" :file-list="videofileList" :action="videoAction"
:before-upload="videoBeforeUpload" name="videofile" accept=".MP4">
<el-button size="small" type="primary" icon="el-icon-upload">点击上传</el-button>
<!-- <div slot="tip" class="el-upload__tip">只能上传不超过200KB的图片文件</div> -->
</el-upload>
</el-form-item>
<el-form-item label="封面图片" prop="videoAddress">
<el-upload ref="pic" :headers="headers" :on-success="urlSuccess" :file-list="videofileList" :action="picAction"
:before-upload="videoBeforeUpload" name="picfile" accept=".jpg">
<el-button size="small" type="primary" icon="el-icon-upload">点击上传</el-button>
<!-- <div slot="tip" class="el-upload__tip">只能上传不超过200KB的图片文件</div> -->
</el-upload>
</el-form-item>
<el-form-item label="视频排序" prop="videoSort">
<el-input v-model="form.videoSort" placeholder="请输入视频排序" oninput="value=value.replace(/[^\d.]/g,'')"></el-input>
</el-form-item>
</el-form>
<div slot="footer">
<el-button @click="submitForm" type="primary">确定</el-button>
<el-button @click="cancel" >取消</el-button>
</div>
</el-dialog>
</div>
</template>
<script>
import {listVideo,addVideo,removeVideo,getVideo,editVideo} from '@/api/basic/video';
import {listWard} from '@/api/basic/ward';
import { getToken } from '@/utils/auth';
export default {
data(){
return{
headers:{
Authorization:"Bearer " + getToken()
},
videofileList:[],
videoAction: process.env.VUE_APP_BASE_API + "/basic/video/video_upload",
picAction: process.env.VUE_APP_BASE_API + "/basic/video/pic_upload",
listWard:[],//病区列表
videoList:[],//视频列表
queryParam:{ //查询参数
video:null, //video名称
wardId:null, //所属病区
wardName:null,//所属病区名称
videoWay:null,//视频路径
videoAddress:null,//封面图片地址
videoSort:null,//视频排序
pageNum:1, //页码
pageSize:10 ,//每页显示的条数
total:0 //总条数
},
title:null,//对话框标题
open:false, //对话框是否显示
//表单内容
form:{
id:null,
video:null, //video名称
wardId:null, //所属病区
wardName:null,//病区名称
videoWay:null,//视频路径
videoAddress:null,//封面图片地址
videoSort:null//视频排序
},
}
},
created(){
this.getList();
this.getWard();
},
methods:{
/**
* 视频上传前的处理
*/
videoBeforeUpload(file){
return true;
},
/**
* 视频上传之后的回调
*/
videoSuccess(response,file, fileList){
console.log(response);
},
/**
* 图片上传前的处理
*/
videoBeforeUpload(file){
return true;
},
/**
* 图片上传之后的回调
*/
urlSuccess(response,file, fileList){
console.log(response);
},
/*查询video列表*/
getList(){
console.log(this.queryParam)
listVideo(this.queryParam).then(response=>{
console.log(response);
this.videoList=response.rows;
this.queryParam.total=response.total;
});
},
/*查询病区列表*/
getWard(){
console.log(this.queryParam)
listWard(this.queryParam).then(response=>{
console.log(response);
this.listWard=response.rows;
this.queryParam.total=response.total;
});
},
/*修改按钮操作 */
handleUpdate(row){
console.log(row)
getVideo({id:row.id}).then(response=>{
console.log(response)
this.form=response.data;
})
this.title="修改视频";
this.open=true;
},
/*删除按钮操作*/
handledelecte(row){
console.log(row)
removeVideo({id:row.id}).then(response=>{
console.log("添加成功")
console.log(response)
if(response.code==200){
this.$modal.msgSuccess(response.msg);
}else{
this.$modal.msgError(response.msg);
}
this.cancel();
this.getList();
})
},
/*新增按钮操作 */
handleAdd(){
this.resetForm("form");//重置表单
this.title="添加视频";
this.open=true;
},
/*按钮取消对话框 */
cancel(){
this.open=false;
},
/*提交按钮操作 */
submitForm(){
console.log("提交成功");
if(this.form.id==null){//添加video
addVideo(this.form).then(response=>{
console.log("添加成功")
console.log(response)
if(response.code==200){
this.$modal.msgSuccess(response.msg);
}else{
this.$modal.msgError(response.msg);
}
this.cancel();
this.getList();
})
}else{//修改video
editVideo(this.form).then(response=>{
if(response.code==200){
this.$modal.msgSuccess(response.msg);
}else{
this.$modal.msgError(response.msg);
}
this.cancel();
this.getList();
})
}
},
handelQuery(){
this.queryParam.pageNum=1;
this.getList();
},
resetQuery(){
this.resetForm("queryForm");
this.handelQuery();
}
}
}
</script>
<style >
</style>
更多推荐
所有评论(0)