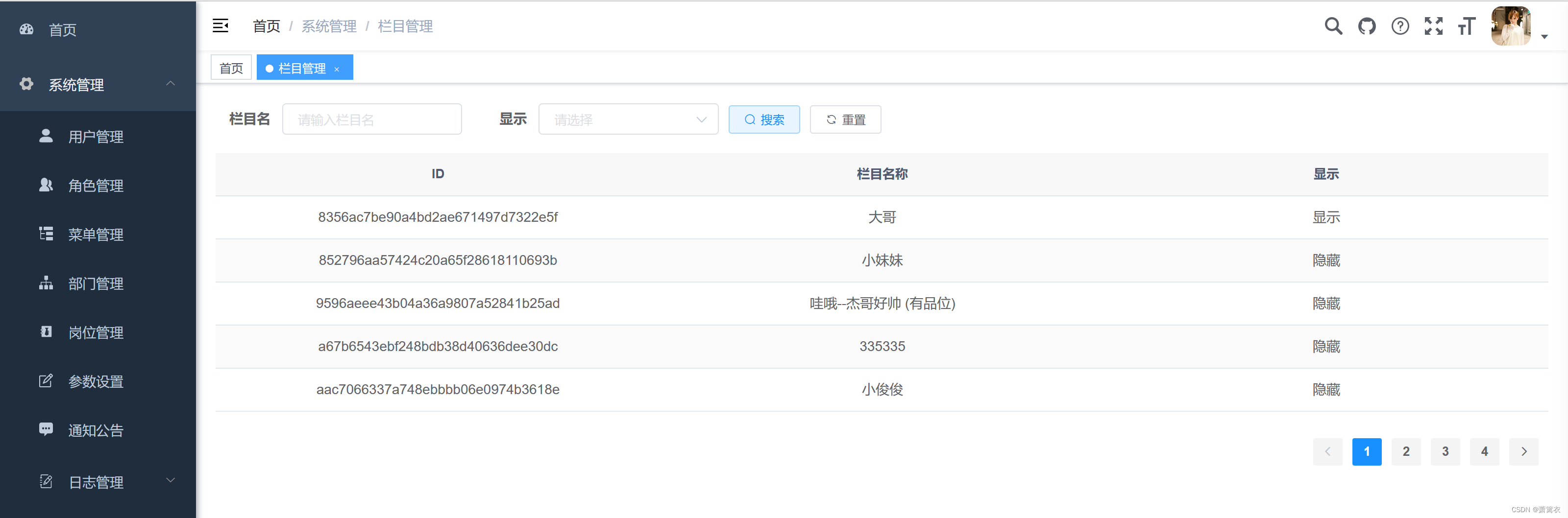
【若依】框架:第02讲前后端分离项目——前端vue
VS Code前端页面编写
·
Icon 图标 (组件) - Element UI 中文开发手册 - 开发者手册 - 腾讯云开发者社区-腾讯云
目标:将数据以表格的形式展现在网页中
一、在VS Code里创建文件
在src-api下新建自己的包basic,文件channel.js,这个js文件的作用就是发送网络请求,相当于uniapp里的uni.request()
channel.js代码如下:
//发送网络请求,相当于uniapp里的uni.request()
import request from '@/utils/request'; //导入网络请求工具
//查询channel列表
export function listChannel(query){
return request({
url: '/basic/channel/list', //请求的路径,controller里的
method: 'get', //请求方式get、post
params: query //请求的参数
});
}
二、页面
在views下新建一个basic文件夹,再建立一个channel文件夹下放index首页
弹框时选择安装,如果没有弹窗可尝试点击其他vue文件,或者在拓展直接搜索下载Vetur安装。
1.搭建框架
<!-- 视图层 -->
<template>
<div>
</div>
</template>
<!-- 业务层 -->
<script>
export default {
//数据(变量的声明)
data(){
return{
}
},
//页面初始化函数,相当于uniapp中的onShow
created(){
},
//函数
methods:{
}
}
</script>
<!-- 样式表 -->
<style>
</style>
2.导入请求后台接口的js文件
// 如果是导入多个函数,在花括号内用逗号隔开即可
import {listChannel} from '@/api/basic/channel';
3.查询channel列表
//数据(变量的声明)
data(){
return{
queryParam: { //查询的参数
channelName: null, //channel名称,和后台项目中channel实体类的属性对应,建议直接复制粘贴
isShow: 0, //是否显示,0=不显示;1显示
//用于分页器的变量
pageNum: 1, //页码
pageSize: 5, //每页显示的条数
total: 0 //总条数
},
}
},
//页面初始化函数,相当于uniapp中的onShow
created(){
this.getList();
},
//函数
methods:{
/*查询channel列表*/
getList(){
listChannel(this.queryParam).then(response => {
console.log(response);
});
}
}
4.打开终端运行(注意后台项目要启动)
首页——菜单管理——系统管理——新增——菜单名称(栏目管理)
新增成功后点击栏目管理看控制台是否有信息输出,获取到代表成功。
5.将数据放入表格中
<el-table :data="channelList" stripe>
<el-table-column label="ID" prop="id" align="center"></el-table-column>
<el-table-column label="栏目名称" prop="channelName" align="center"></el-table-column>
<el-table-column label="显示" prop="isShow" align="center"></el-table-column>
</el-table>
<pagination :page.sync="queryParam.pageNum" :limit.sync="queryParam.pageSize" layout="prev,pager,next" :total="queryParam.total" @pagination="getList"></pagination>
export default {
//数据(变量的声明)
data(){
return{
channelList: null,
queryParam: { //查询的参数
channelName: null, //channel名称,和后台项目中channel实体类的属性对应,建议直接复制粘贴
isShow: null, //是否显示,0=不显示;1显示
//用于分页器的变量
pageNum: 1, //页码
pageSize: 5, //每页显示的条数
total: 0 //总条数
}
}
},
//页面初始化函数,相当于uniapp中的onShow
created(){
this.getList();
},
//函数
methods:{
/*查询channel列表*/
getList(){
listChannel(this.queryParam).then(response => {
console.log(response);
this.channelList = response.rows;
this.queryParam.total = response.total;
});
}
}
}
页面效果如下:
6.搜索重置功能
<el-form :model="queryParam" ref="queryForm" size="small" :inline="true" label-width="68px">
<el-form-item label="栏目名" prop="channelName">
<el-input placeholder="请输入栏目名" v-model="queryParam.channelName" clearable></el-input>
</el-form-item>
<el-form-item label="显示" prop="isShow">
<el-select placeholder="请选择" v-model="queryParam.isShow" size="small">
<el-option v-for="s in showList" :label="s.label" :value="s.value" :key="s.value"></el-option>
</el-select>
</el-form-item>
<el-form-item>
<el-button type="primary" plain @click="handleQuery" size="mini" icon="el-icon-search">搜索</el-button>
<el-button plain @click="resetQuery" size="mini" icon="el-icon-refresh">重置</el-button>
</el-form-item>
</el-form>
<el-table :data="channelList" stripe>
<el-table-column label="ID" prop="id" align="center"></el-table-column>
<el-table-column label="栏目名称" prop="channelName" align="center"></el-table-column>
<el-table-column label="显示" prop="isShow" align="center">
<template slot-scope="scope">
{{scope.row.isShow==0? '隐藏':'显示'}}
</template>
</el-table-column>
</el-table>
<pagination :page.sync="queryParam.pageNum" :limit.sync="queryParam.pageSize" layout="prev,pager,next" :total="queryParam.total" @pagination="getList"></pagination>
<script>
// 如果是导入多个函数,在花括号内用逗号隔开即可
import {listChannel} from '@/api/basic/channel'; //导入请求后台接口的js文件
export default {
//数据(变量的声明)
data(){
return{
channelList: null,
queryParam: { //查询的参数
channelName: null, //channel名称,和后台项目中channel实体类的属性对应,建议直接复制粘贴
isShow: null, //是否显示,0=不显示;1显示
//用于分页器的变量
pageNum: 1, //页码
pageSize: 5, //每页显示的条数
total: 0 //总条数
},
//下拉列表中要显示的内容
showList:[
{label:'隐藏',value:0},
{label:'显示',value:1}
]
}
},
//页面初始化函数,相当于uniapp中的onShow
created(){
this.getList();
},
//函数
methods:{
/*查询channel列表*/
getList(){
listChannel(this.queryParam).then(response => {
console.log(response);
this.channelList = response.rows;
this.queryParam.total = response.total;
});
},
// 表格数据条件搜索
handleQuery(){
this.queryParam.pageNum = 1; //给分页器的页码归位
this.getList();
},
//重置搜索表单
resetQuery(){
this.resetForm("queryForm"); //重置表单
this.handleQuery(); //刷新请求
}
}
}
</script>
页面如下:
完整前端代码如下:
<!-- 视图层 -->
<template>
<div class="app-container">
<el-form :model="queryParam" ref="queryForm" size="small" :inline="true" label-width="68px">
<el-form-item label="栏目名" prop="channelName">
<el-input placeholder="请输入栏目名" v-model="queryParam.channelName" clearable></el-input>
</el-form-item>
<el-form-item label="显示" prop="isShow">
<el-select placeholder="请选择" v-model="queryParam.isShow" size="small">
<el-option v-for="s in showList" :label="s.label" :value="s.value" :key="s.value"></el-option>
</el-select>
</el-form-item>
<el-form-item>
<el-button type="primary" plain @click="handleQuery" size="mini" icon="el-icon-search">搜索</el-button>
<el-button plain @click="resetQuery" size="mini" icon="el-icon-refresh">重置</el-button>
</el-form-item>
</el-form>
<el-table :data="channelList" stripe>
<el-table-column label="ID" prop="id" align="center"></el-table-column>
<el-table-column label="栏目名称" prop="channelName" align="center"></el-table-column>
<el-table-column label="显示" prop="isShow" align="center">
<template slot-scope="scope">
{{scope.row.isShow==0? '隐藏':'显示'}}
</template>
</el-table-column>
</el-table>
<pagination :page.sync="queryParam.pageNum" :limit.sync="queryParam.pageSize" layout="prev,pager,next" :total="queryParam.total" @pagination="getList"></pagination>
</div>
</template>
<!-- 业务层 -->
<script>
// 如果是导入多个函数,在花括号内用逗号隔开即可
import {listChannel} from '@/api/basic/channel'; //导入请求后台接口的js文件
export default {
//数据(变量的声明)
data(){
return{
channelList: null,
queryParam: { //查询的参数
channelName: null, //channel名称,和后台项目中channel实体类的属性对应,建议直接复制粘贴
isShow: null, //是否显示,0=不显示;1显示
//用于分页器的变量
pageNum: 1, //页码
pageSize: 5, //每页显示的条数
total: 0 //总条数
},
//下拉列表中要显示的内容
showList:[
{label:'隐藏',value:0},
{label:'显示',value:1}
]
}
},
//页面初始化函数,相当于uniapp中的onShow
created(){
this.getList();
},
//函数
methods:{
/*查询channel列表*/
getList(){
listChannel(this.queryParam).then(response => {
console.log(response);
this.channelList = response.rows;
this.queryParam.total = response.total;
});
},
// 表格数据条件搜索
handleQuery(){
this.queryParam.pageNum = 1; //给分页器的页码归位
this.getList();
},
//重置搜索表单
resetQuery(){
this.resetForm("queryForm"); //重置表单
this.handleQuery(); //刷新请求
}
}
}
</script>
<!-- 样式表 -->
<style>
</style>
更多推荐
所有评论(0)